Top Python Interview Questions And Answers For 2024
Prepare for your Python interviews with these top Python interview questions and answers for 2024, designed for programmers.
Landing your dream data science job requires excelling not only in technical skills but also in demonstrating your ability to translate those skills into practical solutions.This blog post equips you with the knowledge and strategies to confidently tackle a crucial aspect of the data science interview process: Python coding interview questions.
We'll delve into various difficulty levels, from fundamental syntax challenges to implementing advanced algorithms. But before we dive into the code, let's explore some general tips for preparing for data science interviews. Here are a few key areas to focus on:
- Research the company and role: Understanding their work and the specific requirements of the position allows you to tailor your responses and showcase your relevant skills.
- Brush up on data science fundamentals: Revisit core concepts like statistics, machine learning, and data wrangling.
- Practice problem-solving: Regularly challenge yourself with coding problems on platforms like LeetCode or HackerRank. This sharpens your problem-solving skills and ability to think critically under pressure.
- Prepare for behavioral questions: Be ready to articulate your past experiences using the STAR method (Situation, Task, Action, Result) to demonstrate your skills and approach to problem-solving.
By following these tips and mastering the Python coding interview questions covered in this guide, you'll significantly enhance your confidence and preparation for your data science interview. Let's get started!
Focus Areas for Python Interviews
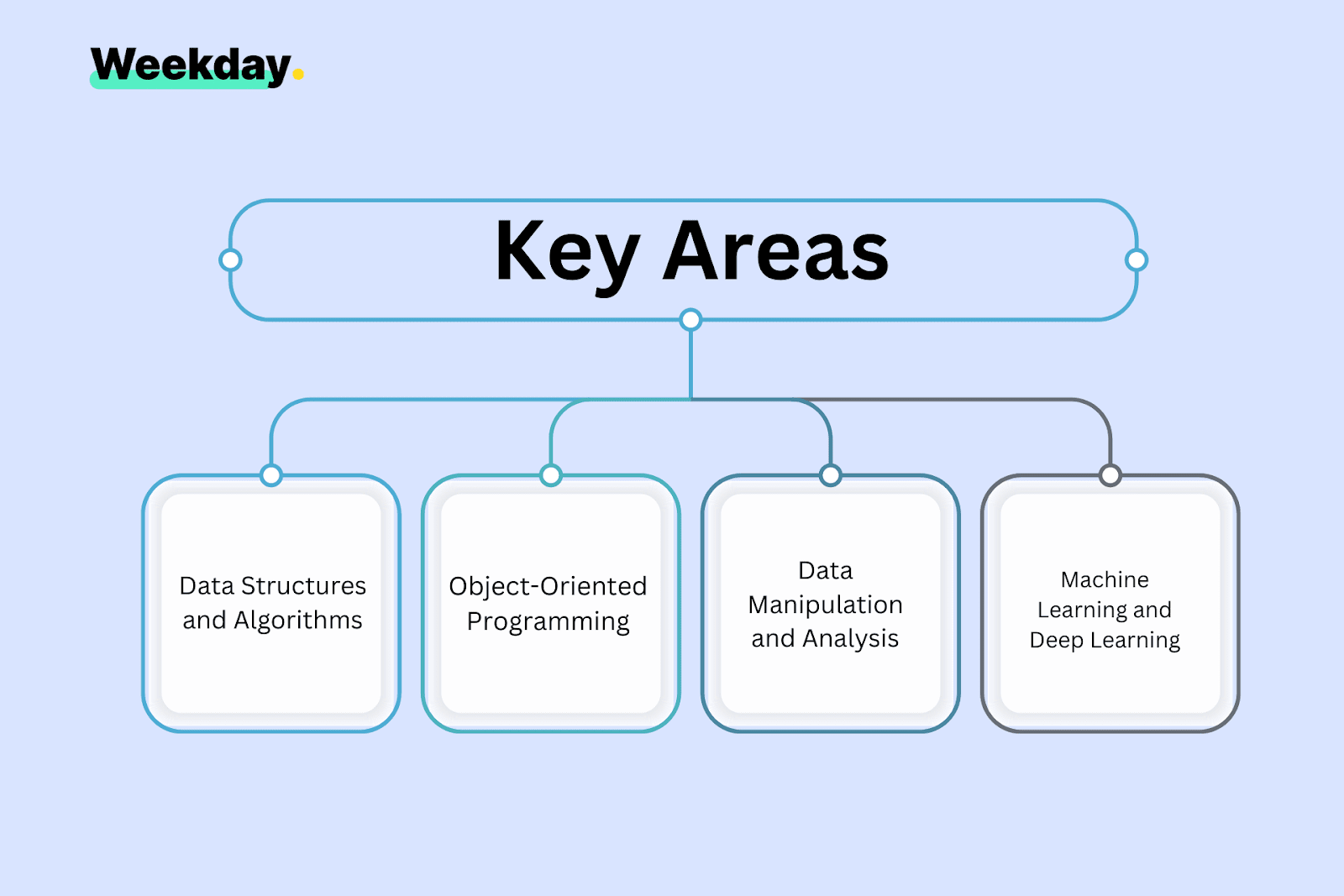
During your interview, expect questions that assess your competency in these key areas:
- Data Structures and Algorithms: Understanding how to store, organize, and manipulate data efficiently is fundamental. Be prepared to discuss concepts like lists, dictionaries, sets, and common algorithms for sorting, searching, and filtering data.
- Object-Oriented Programming (OOP): OOP principles like classes, objects, inheritance, and polymorphism are essential for building modular and reusable code. Demonstrate your grasp of these concepts and how you'd apply them to data science projects.
- Data Manipulation and Analysis: Your ability to clean, transform, and analyze data using Python libraries like Pandas will be heavily tested. Be prepared to showcase your proficiency in data wrangling techniques.
- Machine Learning and Deep Learning: Companies often gauge your understanding of core machine learning concepts like linear regression, decision trees, and random forests. Additionally, knowledge of deep learning frameworks like TensorFlow or PyTorch could be advantageous.
Python Interview Questions: Coding
Now, let's delve into the nitty-gritty of Python coding interview questions:
Basic Python Coding:
Question 1: Write a Python program to check if a number is even or odd.
Answer:
def is_even(number):
return number % 2 == 0
# Example usage
number = 10
if is_even(number):
print(f"{number} is even.")
else:
print(f"{number} is odd.")
Question 2: Write a Python program to find the factorial of a number.
Answer:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
# Example usage
number = 5
result = factorial(number)
print(f"The factorial of {number} is {result}.")
This code defines a function factorial that uses recursion to calculate the factorial of a number. The factorial of a number is the product of all positive integers less than or equal to that number. The base case is when n is 0, where the factorial is 1. For other cases, the function calls itself recursively with n-1 and multiplies the result by n.
Question 3: Write a Python program to find the largest number in a list.
Answer:
def find_largest(numbers):
largest = numbers[0]
for number in numbers:
if number > largest:
largest = number
return largest
# Example usage
numbers = [3, 7, 1, 9, 4]
largest_number = find_largest(numbers)
print(f"The largest number in the list is {largest_number}.")
This code defines a function find_largest that iterates through a list of numbers and keeps track of the largest number encountered so far. It returns the largest number at the end.
Question 4: Write a Python program to convert a string to uppercase and lowercase.
Answer:
text = "Hello, World!"
uppercase_text = text.upper()
lowercase_text = text.lower()
print(f"Uppercase: {uppercase_text}")
print(f"Lowercase: {lowercase_text}")
Question 5: Write a Python program to find the sum and average of elements in a list.
Answer:
numbers = [1, 2, 3, 4, 5]
total_sum = sum(numbers)
average = total_sum / len(numbers)
print(f"Sum: {total_sum}")
print(f"Average: {average:.2f}") # Format average to two decimal places
Question 6: Write a Python program to check if a string contains a specific substring.
Answer:
text = "This is a string"
substring = "is"
if substring in text:
print(f"The string '{substring}' is found in '{text}'.")
else:
print(f"The string '{substring}' is not found in '{text}'.")
Question 7: Write a Python program to print the elements of a list in reverse order.
Answer:
numbers = [1, 2, 3, 4, 5]
# Method 1: Using a reversed loop
for num in reversed(numbers):
print(num)
# Method 2: Slicing with a negative step
print(numbers[::-1]) # Prints a reversed copy of the list
Question 8: Write a Python program to remove duplicate elements from a list.
Answer:
numbers = [1, 2, 2, 3, 4, 4, 5]
# Method 1: Using set conversion (removes duplicates by default)
unique_numbers = set(numbers)
print(f"Unique elements: {list(unique_numbers)}") # Convert back to list for printing
# Method 2: Using a loop and conditional check (less memory efficient)
unique_numbers = []
for num in numbers:
if num not in unique_numbers:
unique_numbers.append(num)
print(f"Unique elements: {unique_numbers}")
Question 9: Write a Python program to check if a character is a vowel or consonant.
Answer:
def is_vowel(char):
"""Checks if a character is a vowel (case-insensitive)."""
vowels = "aeiouAEIOU"
return char in vowels
character = input("Enter a character: ")
if is_vowel(character):
print(f"{character} is a vowel.")
Else:
print(f"{character} is a consonant.")
Question 10: Write a Python program to find the length of a string.
Answer:
text = "This is a string"
length = len(text)
print(f"Length of the string: {length}")
Python Interview Questions: Intermediate Coding
Question 11: Find the minimum and maximum elements in a list:
Answer:
numbers = [1, 5, 2, 8, 3]
min_number = min(numbers)
max_number = max(numbers)
print(f"Minimum: {min_number}")
print(f"Maximum: {max_number}")
Question 12: Filter elements from a list based on a condition:
Answer:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [num for num in numbers if num % 2 == 0] # List comprehension for even numbers
print(f"Even numbers: {even_numbers}")
Question 13: Create a dictionary from a list of key-value pairs:
Answer:
key_value_pairs = [("name", "Alice"), ("age", 30), ("city", "New York")]
my_dict = dict(key_value_pairs)
print(f"Dictionary: {my_dict}")
Question 14: Write a Python program to iterate over a dictionary and perform operations on key-value pairs.
Answer:
fruits = {"apple": 2, "banana": 3, "cherry": 1}
total_quantity = 0
for fruit, quantity in fruits.items():
print(f"{fruit}: {quantity}")
total_quantity += quantity
print(f"Total quantity: {total_quantity}")
Question 15: Write a Python program to sort a list in ascending or descending order.
Answer:
numbers = [5, 2, 8, 1, 4]
# Sort in ascending order (default)
sorted_numbers = sorted(numbers)
# Sort in descending order (reverse=True)
reversed_sorted_numbers = sorted(numbers, reverse=True)
print(f"Ascending order: {sorted_numbers}")
print(f"Descending order: {reversed_sorted_numbers}")
Question 16: Write a Python program to create a simple function that takes arguments and performs calculations.
Answer:
def calculate_area(length, width):
"""Calculates the area of a rectangle."""
return length * width
# Example usage
area = calculate_area(5, 3)
print(f"Area of rectangle: {area}")
Question 17: Write a Python program to implement a simple text-based menu using loops and conditional statements.
Answer:
def main():
while True:
print("Menu:")
print("1. Add numbers")
print("2. Subtract numbers")
print("3. Exit")
choice = input("Enter your choice (1-3): ")
if choice == '1':
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
result = num1 + num2
print(f"Sum: {result}")
elif choice == '2':
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
result = num1 - num2
print(f"Difference: {result}")
elif choice == '3':
print("Exiting...")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
Question 18: Write a Python program to check if a string is a palindrome.A palindrome is a word or phrase that reads the same backward as forward (e.g., "racecar", "madam").
Answer:
def is_palindrome(text):
"""Checks if a string is a palindrome (case-insensitive)."""
text = text.lower().replace(" ", "") # Remove spaces and convert to lowercase
return text == text[::-1]
# Example usage
text = "Race car"
if is_palindrome(text):
print(f"{text} is a palindrome.")
else:
print(f"{text} is not a palindrome.")
Question 19: Write two Python programs, one using a loop and another using list comprehension, to achieve the same functionality of squaring all the elements in a list.
Answer:
Solution 1: Using a Loop
def square_list_loop(numbers):
"""Squares all elements in a list using a loop."""
squared_numbers = []
for number in numbers:
squared_numbers.append(number * number)
return squared_numbers
# Example usage
numbers = [1, 2, 3, 4, 5]
squared_numbers = square_list_loop(numbers)
print(f"Squared list (loop): {squared_numbers}")
Question 20: Write a Python program to find the second largest element in a list.
Answer:
Solution 1: Using Sorting and Indexing
def find_second_largest_sorted(numbers):
"""Finds the second largest element using sorting and indexing."""
if len(numbers) < 2:
return None # Handle cases with less than 2 elements
# Sort the list in descending order
sorted_numbers = sorted(numbers, reverse=True)
# Return the second element (assuming no duplicates)
return sorted_numbers[1]
# Example usage
numbers = [5, 8, 2, 9, 1]
second_largest = find_second_largest_sorted(numbers)
print(f"Second largest (sorted): {second_largest}")
Advanced Python Interview Questions Coding:
Here are 10 advanced Python coding interview questions and answers to challenge your skills:
Question 21: Write a Python program to implement a recursive function for calculating the factorial of a number.
Answer:
def factorial(n):
"""Calculates the factorial of a number recursively."""
if n == 0:
return 1
else:
return n * factorial(n-1)
# Example usage
number = 5
result = factorial(number)
print(f"Factorial of {number} is {result}.")
Question 22: Write a Python program to implement a function for finding the GCD (Greatest Common Divisor) of two numbers.
Answer:
def gcd(a, b):
"""Calculates the Greatest Common Divisor of two numbers recursively."""
if b == 0:
return a
else:
return gcd(b, a % b)
# Example usage
x = 18
y = 12
gcd_value = gcd(x, y)
print(f"GCD of {x} and {y} is {gcd_value}.")
Question 23: Write a Python program to implement a function for checking if a string is a valid parenthesis expression.
Answer:
def is_valid_parenthesis(expression):
"""Checks if a string is a valid parenthesis expression."""
stack = []
mapping = {"(": ")", "{": "}", "[": "]"}
for char in expression:
if char in mapping:
stack.append(char)
elif char in mapping.values():
if not stack or mapping[stack.pop()] != char:
return False
return not stack
# Example usage
expression = "([)]"
if is_valid_parenthesis(expression):
print(f"{expression} is a valid parenthesis expression.")
else:
print(f"{expression} is not a valid parenthesis expression.")
Question 24: Write a Python program to implement a function for finding the longest common substring in two strings.
Answer:
def longest_common_substring(text1, text2):
"""Finds the longest common substring in two strings."""
m = len(text1)
n = len(text2)
dp = [[0 for _ in range(n + 1)] for _ in range(m + 1)]
# Find the length of the longest common substring
longest = 0
for i in range(1, m + 1):
for j in range(1, n + 1):
if text1[i - 1] == text2[j - 1]:
dp[i][j] = dp[i - 1][j - 1] + 1
longest = max(longest, dp[i][j])
else:
dp[i][j] = 0
# Construct the longest common substring based on the dp table (optional)
substring = ""
i = m
j = n
while i > 0 and j > 0:
if dp[i][j] > 0:
substring = text1[i - 1] + substring
i -= 1
j -= 1
else:
break
return substring
# Example usage
text1 = "Geeks
Question 25: Write a Python program to implement a function for performing memoization on a function.
Answer:
def memoize(func):
"""Memoizes a function to store and reuse results."""
cache = {}
def memoized_func(*args):
if args in cache:
return cache[args]
else:
result = func(*args)
cache[args] = result
return result
return memoized_func
@memoize
def fibonacci(n):
"""Calculates the nth Fibonacci number (example function to memoize)."""
if n == 0 or n == 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# Example usage
result = fibonacci(35) # Memoization avoids redundant calculations
print(f"Fibonacci of 35: {result}")
Question 26: Write a Python program to implement a custom data structure like a binary search tree (BST).
Answer:
class Node:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, data):
# Implementation for inserting a node into the BST
def search(self, data):
# Implementation for searching a node in the BST
def delete(self, data):
# Implementation for deleting a node from the BST
# Example usage (demonstration omitted for brevity)
Question 27: Write a Python program to implement a function for parsing a JSON string and converting it into a Python dictionary.
Answer:
import json
def parse_json(json_string):
"""Parses a JSON string and converts it into a Python dictionary."""
try:
data = json.loads(json_string)
return data
except json.JSONDecodeError as e:
print(f"Error parsing JSON: {e}")
return None
# Example usage
json_data = '{"name": "Alice", "age": 30}'
data_dict = parse_json(json_data)
print(f"Parsed data: {data_dict}")
Question 28: Write a Python program to implement a multithreaded application using the threading module.
Answer:
import threading
def worker(name):
"""Simulates a worker function."""
print(f"Worker {name} started")
# Simulate some work (replace with your actual task)
for i in range(3):
print(f"Worker {name} doing some work - {i}")
print(f"Worker {name} finished")
# Create and start two worker threads
threads = []
for i in range(2):
thread = threading.Thread(target=worker, args=(f"Thread-{i+1}",))
threads.append(thread)
thread.start()
# Wait for all threads to finish (optional)
for thread in threads:
thread.join()
print("All workers finished!")
Question 29: Write a Python program to implement a web scraper using a library like BeautifulSoup to extract data from a website.
Answer:
import requests
from bs4 import BeautifulSoup
def scrape_website(url):
"""Scrapes data from a website using BeautifulSoup."""
try:
response = requests.get(url)
response.raise_for_status() # Raise exception for non-200 status codes
soup = BeautifulSoup(response.content, 'html.parser')
# Example: Extract all titles from h1 tags
titles = []
for h1 in soup.find_all('h1'):
titles.append(h1.text.strip())
return titles
except requests.exceptions.RequestException as e:
print(f"Error scraping website: {e}")
return None
# Example usage
url = "https://www.example.com" # Replace with the target website
extracted_titles = scrape_website(url)
if extracted_titles:
print(f"Extracted titles: {extracted_titles}")
else:
print("Failed to scrape website.")
Question 30: Write a Python program to implement a function for finding the minimum edit distance between two strings.
Answer:
def minimum_edit_distance_dp(str1, str2):
"""Finds the minimum edit distance between two strings using dynamic programming."""
m = len(str1) + 1
n = len(str2) + 1
# Create a table to store minimum edit distances for subproblems
dp = [[0 for _ in range(n)] for _ in range(m)]
# Fill the base cases (empty strings)
for i in range(m):
dp[i][0] = i # i insertions needed to transform an empty string to str1[0:i]
for j in range(n):
dp[0][j] = j # j insertions needed to transform an empty string to str2[0:j]
# Fill the remaining table cells
for i in range(1, m):
for j in range(1, n):
if str1[i - 1] == str2[j - 1]:
# No edit needed if characters are the same
dp[i][j] = dp[i - 1][j - 1]
else:
# Minimum of (insert, delete, substitute) operations
insertion = dp[i][j - 1] + 1
deletion = dp[i - 1][j] + 1
substitution = dp[i - 1][j - 1] + 1
dp[i][j] = min(insertion, deletion, substitution)
return dp[m - 1][n - 1] # Minimum edit distance at the bottom right corner
# Example usage
str1 = "kitten"
str2 = "sitting"
distance = minimum_edit_distance_dp(str1, str2)
print(f"Minimum edit distance between '{str1}' and '{str2}': {distance}")
Reading List
This section provides a curated list of resources to expand your Python knowledge and explore advanced topics:
Books:
- "Python Crash Course" by Eric Matthes: A comprehensive introduction to Python, covering programming fundamentals, data structures, algorithms, and object-oriented programming.
- "Hands-On Machine Learning with Scikit-Learn, Keras & TensorFlow" by Aurélien Géron: A practical guide to building machine learning models using popular Python libraries.
- "Data Science for Business" by Foster Provost and Tom Fawcett: Explores the core concepts of data science and how Python is used to extract insights from data.
Also Read: Top React Native Interview Questions
Online Courses That Helps In Python Interview Questions
- "Coursera - Python for Everybody Specialization:" by University of Michigan: A well-structured program covering Python basics, data structures, and programming techniques.
- "DataCamp - Introduction to Python for Data Science”:
Learn Python fundamentals tailored for data science applications, including data manipulation and visualization. - "edX - Introduction to Computer Science and Programming Using Python: A broad introduction to computer science concepts using Python, covering programming paradigms and problem-solving approaches.
Documentation and Tutorials:
- Official Python Tutorial: The official Python documentation offers a comprehensive guide to the language, from syntax to advanced features.
- NumPy Documentation: Detailed documentation for NumPy, a fundamental library for numerical computing in Python.
- Pandas Documentation: Provides extensive documentation for Pandas, a powerful library for data manipulation and analysis.
Remember, this list is just a starting point. Explore other resources that pique your interest and align with your learning goals. By actively engaging with these materials and practicing your coding skills, you'll continuously enhance your Python proficiency.
Conclusion
This guide has served as a roadmap for navigating Python interview questions commonly encountered in data science recruitment. It has explored various difficulty levels, from basic syntax challenges to advanced algorithms. By diligently practicing with the provided examples and venturing into the resources within the Reading List, you'll be well-equipped to showcase your Python expertise and confidently tackle your data science job interview.
Recap and Encouragement for Further Learning and Practice
Let's solidify your learnings and propel you further on your data science journey! Here are some key takeaways and pointers for continuous learning and effective practice:
- Master the fundamentals: Revisit the core Python concepts covered in this guide and ensure a solid understanding of syntax, data structures, and algorithms.
- Embrace active practice: Regularly challenge yourself with coding problems on platforms like LeetCode or HackerRank.
- Diversify your practice: Explore different problem types and difficulty levels to broaden your skills and adaptability.
- Don't be afraid to experiment: Try different approaches to problems and analyze their efficiency and effectiveness.
- Seek out new challenges: Participate in online coding competitions or contribute to open-source projects to gain real-world experience.
- Never stop learning: The world of Python and data science is constantly evolving. Explore the Reading List resources and delve deeper into concepts that pique your interest. Stay updated with the latest trends and libraries to remain competitive.
By actively engaging with these practices and fostering a continuous learning mindset, you'll transform yourself into a highly proficient Python programmer, ready to excel in the dynamic field of data science.
Don't stop here! The data science field thrives on continuous learning. Explore the vast possibilities of Python and delve deeper into concepts that spark your curiosity. As you refine your skills, visit the candidate page on our weekday portal to discover new job opportunities and see how your Python mastery can translate into real-world data science applications. We believe in your potential and are committed to supporting your growth in the exciting world of data science!