Introduction: Ace Your Node.js Interview Questions and Answers
The Node.js developer interview looms – a chance to showcase your skills and land your dream job. But with a vast array of potential questions, where do you begin? Don't sweat it! This guide equips you with the arsenal you need to excel in the interview.
We'll provide the most frequently asked Node.js interview questions with detailed answers, unraveling core concepts and advanced techniques. You'll emerge confident, ready to impress with your problem-solving prowess and clear communication.
Get ready to:
- Master asynchronous programming challenges with clear explanations and code examples.
- Deep dive into popular frameworks like Express.js, expanding your developer skillset.
- Shine during the interview by showcasing your ability to solve problems and communicate effectively.
By the end of this journey, you'll be well-prepared to land that coveted Node.js developer role.
Ready to transform interview jitters into interview triumph? Let's begin!
Node.js Fundamentals for Interviews

This section focuses on equipping you with a solid understanding of fundamental Node.js concepts interviewers frequently assess.
1. Event Loop and Non-Blocking I/O:
- Question: Explain the concept of the event loop in Node.js and how it enables non-blocking I/O operations.
- Answer: Node.js operates on a single-threaded event loop. This means it can only execute one task at a time. However, the magic lies in its non-blocking I/O operations. When a non-blocking operation like a network request is initiated, the event loop doesn't wait for it to complete. Instead, it moves on to the next task in the queue. Once the I/O operation finishes, the event loop triggers a callback function to handle the result. This allows Node.js to handle multiple concurrent requests efficiently, making it ideal for real-time applications.
Here's a simplified analogy: Imagine you're a chef (the event loop) juggling multiple orders (tasks). If an order requires waiting for the oven (blocking I/O), you wouldn't wait idly - you'd move on to preparing another dish (non-blocking I/O). Once the oven is done, you'd be notified (callback function) to plate and deliver the dish.
Here is a flowchart to better understand:
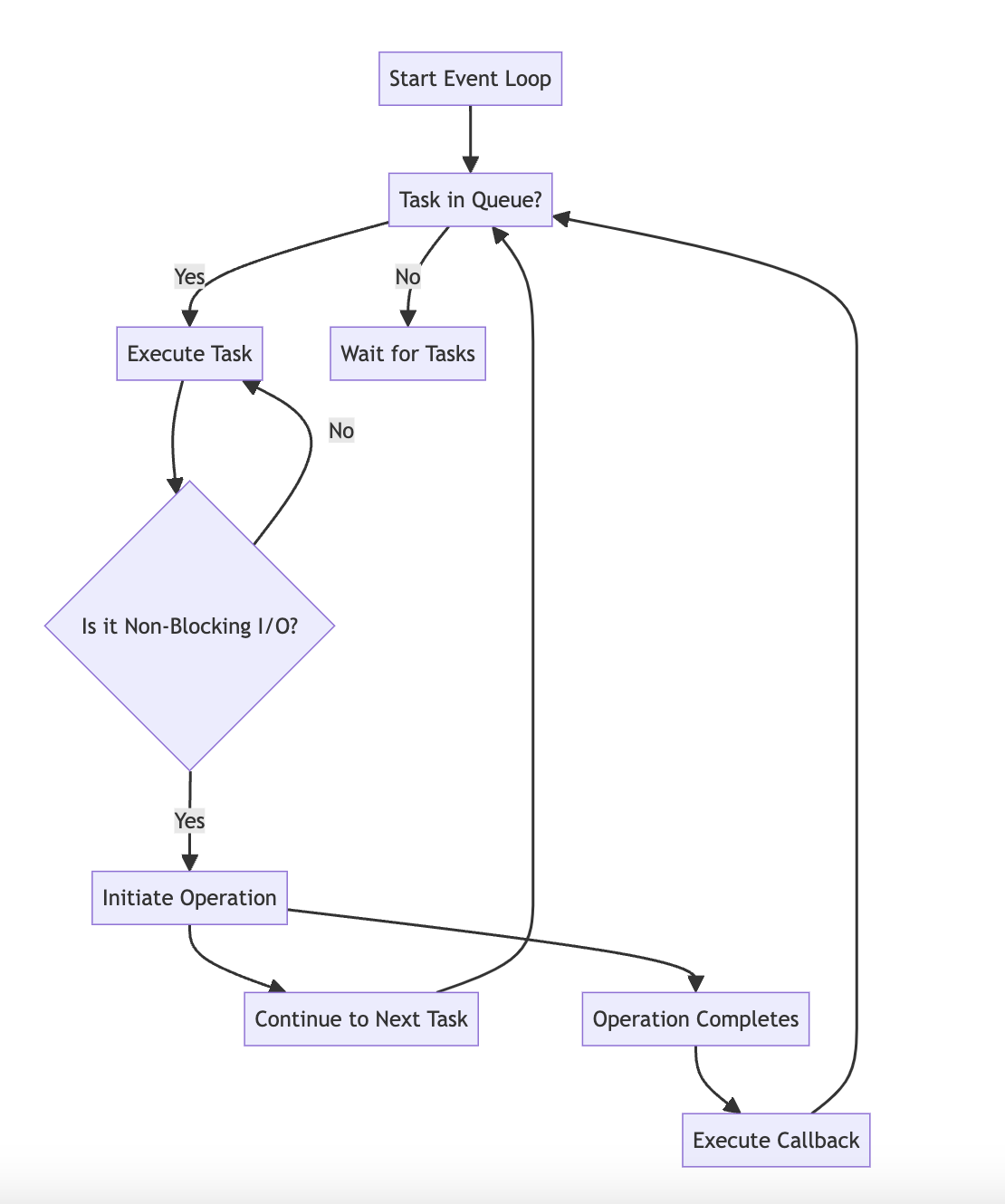
2. Modules and Package Management (npm)
- Question: How do you manage dependencies in a Node.js project using npm?
- Answer: Node.js applications often rely on reusable code modules. This is where npm, the Node Package Manager, comes in handy. It allows you to easily install, manage, and share code modules from a vast public repository called the npm registry.
Here are some key npm commands for managing dependencies:
Table 1: Essential npm Commands
By effectively using npm, you can efficiently manage dependencies, ensuring your project leverages the power of reusable code modules.
We'll continue building upon these fundamentals in the next sections, delving deeper into advanced concepts and interview-worthy scenarios.
3. Asynchronous Programming with Callbacks and Promises
- Question: Explain the difference between callbacks and promises for handling asynchronous operations in Node.js.
- Answer: Both callbacks and promises are mechanisms for dealing with asynchronous operations, but they differ in approach:some text
- Callbacks: The traditional method involves passing a callback function as an argument to the asynchronous function. This function is then invoked once the operation finishes, receiving the result as an argument. While callbacks are simple, nested callbacks can lead to "callback hell," making code difficult to read and maintain.
- Promises: Promises offer a more structured and cleaner approach. They represent the eventual completion (or failure) of an asynchronous operation. You can chain promises together to handle complex asynchronous workflows more elegantly. Promises provide methods like .then() and .catch() for handling successful results and errors, respectively.
4. Error Handling:
- Question: Describe strategies for implementing proper error handling in Node.js applications.
- Answer: Robust error handling is essential for building reliable Node.js applications. Here are some key strategies:some text
- Try...Catch Blocks: Use try...catch blocks to catch potential errors thrown during code execution. The catch block allows you to handle the error gracefully, preventing your application from crashing. You can also include a finally block to execute code regardless of whether an error occurs.
- Async/Await Error Handling: When using async/await for asynchronous operations, you can handle errors using a try...catch block within the async function. This simplifies error handling compared to traditional callbacks.
5. Streams:
- Question: Explain the concept of streams in Node.js and their role in data processing.
- Answer: Streams provide a powerful mechanism for handling data in a continuous flow. They represent a sequence of data chunks that can be read from (readable streams) or written to (writable streams). Node.js offers various built-in stream types:some text
- Readable Streams: Emit data chunks that can be processed piece by piece. Examples include file reading streams or network response streams.
- Writable Streams: Allow you to write data chunks sequentially. Examples include file writing streams or network request streams.
- Duplex Streams: Combine both readable and writable capabilities, enabling two-way data flow. An example is a TCP socket stream.
6. Timers:
- Question: How do you use timers in Node.js to schedule tasks at specific times or intervals?
- Answer: Node.js provides several timer functions for scheduling tasks:some text
- setTimeout(callback, delay, [arg1, arg2, ...]): Schedules a callback function to be executed after a specified delay (in milliseconds). Optional arguments can be passed to the callback function.
- setInterval(callback, delay, [arg1, arg2, ...]): Schedules a callback function to be executed repeatedly, with a fixed time interval between each execution.
- clearTimeout(timeoutId): Clears a previously set timeout timer identified by its ID.
- clearInterval(intervalId): Clears a previously set interval timer identified by its ID.
7. Buffers:
- Question: Explain the concept of Buffers in Node.js and their use for binary data.
- Answer: Buffers are used to represent raw binary data in Node.js. They provide an efficient way to handle data streams like network requests or file I/O that often deal with binary content. Buffers offer methods for manipulating binary data, such as reading, writing, and copying bytes.
8. The global Object:
- Question: Discuss the global object in Node.js and why it's generally discouraged to use it excessively.
- Answer: The global object provides a global namespace in Node.js. However, it's important to understand how variables declared outside of functions behave:
- In browsers: Variables declared with var outside of functions become global properties accessible from anywhere in the code.
- In Node.js: Variables declared with var outside of functions are added to the module scope, not the global scope. This means they are only accessible within the current module file.
While you can still access the global object and assign variables to it, excessive reliance on it is discouraged for the following reasons:
- Naming conflicts: Global variables can lead to naming conflicts, especially when working on larger projects or collaborating with others.
- Maintainability concerns: Code that heavily relies on global variables becomes harder to understand and maintain. It's difficult to track where a variable is defined and used throughout the application.
For better code organization and encapsulation, it's generally recommended to use:
- Local variables within functions
- Function parameters
- Modules to export and import variables between files
Advanced Node.js Concepts for Interviews

Now that you've mastered the fundamentals, let's dive into some advanced Node.js concepts that will truly impress interviewers:
1. The File System (fs) Module:
- Question: Describe how to use the fs module for file system operations in Node.js.
- Answer: The fs module provides a rich set of functions for interacting with the file system. Here are some common operations:some text
- Reading Files:some text
- fs.readFileSync(path, encoding): Reads a file synchronously, returning the entire file contents as a string (optional encoding specified).
- fs.readFile(path, encoding, callback): Reads a file asynchronously, invoking the callback function with the file contents (as a string) or an error upon completion.
- Writing Files:some text
- fs.writeFileSync(path, data, encoding): Writes data synchronously to a file (encoding can be specified).
- fs.writeFile(path, data, encoding, callback): Writes data asynchronously to a file, invoking the callback function upon completion or error.
- Reading Files:some text
Note: While synchronous methods like readFileSync are simpler, they can block the event loop, impacting performance. It's generally recommended to use asynchronous methods like readFile for better responsiveness.
2. HTTP Module and Building a Simple Server:
- Question: Explain the core functionalities of the HTTP module and how to create a basic Node.js HTTP server.
- Answer: The http module is fundamental for building web servers in Node.js. Here's a breakdown of creating a simple server:some text
- Require the http module: javascript const http = require('http');
- Create a server: javascript const server = http.createServer((req, res) => { // Handle incoming requests (req) and send responses (res) });
- Define request handler function:
- This function handles incoming requests, processes them, and sends responses. You can serve static content, handle API requests, or perform more complex logic.
- Start the server: javascript server.listen(port, hostname, () => { console.log(`Server listening on port ${port}`); });
3. Popular Node.js Frameworks (Express.js, etc.):
- Question: Discuss the benefits of using frameworks like Express.js in Node.js development and their key features.
- Answer: While you can build web applications with the core Node.js modules, frameworks like Express.js offer a higher level of abstraction and simplification. Here's why they're valuable:some text
- Routing: Express.js provides a robust routing mechanism for handling different URL paths and HTTP methods (GET, POST, PUT, etc.).
- Middleware: Middleware functions allow you to intercept and process requests before they reach the final handler, enabling features like authentication, logging, and more.
- Templating Engines: Express.js integrates with popular templating engines like EJS or Pug, simplifying dynamic content generation in your web pages.
4. Working with Databases (Mongoose, etc.):
- Question: Explain how to interact with databases from a Node.js application using an Object Document Mapper (ODM) like Mongoose.
- Answer: Node.js applications often interact with databases to store and retrieve data. Mongoose is a popular ODM that simplifies working with MongoDB, a NoSQL database. It allows you to define data models that map to MongoDB collections and provides methods for CRUD (Create, Read, Update, Delete) operations on your data.
5. Testing in Node.js:
- Question: Describe your approach to testing Node.js applications and the importance of writing unit tests.
- Answer: Writing unit tests is crucial for ensuring the reliability and maintainability of your Node.js code. Popular testing frameworks like Jest or Mocha allow you to create unit tests that isolate and test individual functions or modules. This helps catch bugs early in the development process and ensures your code functions as expected.
6. Security Considerations in Node.js:
- Question: Discuss some key security considerations when building Node.js applications.
- Answer: Security is paramount in web development. Here are some essential aspects to consider for Node.js applications:some text
- Input Validation: Sanitize and validate all user input to prevent attacks like SQL injection or cross-site scripting (XSS).
- Authentication and Authorization: Implement robust
Now that you've mastered the fundamentals, let's dive into some advanced Node.js concepts that will truly impress interviewers.
Deep Dive into Advanced Node.js Interview Scenarios
We've covered a range of advanced Node.js concepts that will impress interviewers. Now, let's explore some in-depth interview scenarios that test your problem-solving and analytical skills:
Scenario 1: Scaling a Node.js Application
- Prompt: You're developing a real-time chat application using Node.js, and it's experiencing a surge in user traffic. How would you approach scaling the application to handle the increased load?
- Answer: There are several strategies for scaling a Node.js application:some text
- Horizontal Scaling: Add more worker processes (instances) running on different servers to distribute the workload across multiple machines. This improves concurrency and handles more concurrent requests.
- Load Balancing: Implement a load balancer to distribute incoming traffic evenly across multiple worker processes, optimizing resource utilization.
- Clustering: Use the built-in Node.js cluster module to spawn multiple worker processes on a single server, sharing the same event loop for communication.
- Caching: Implement caching mechanisms to store frequently accessed data in memory, reducing database load and improving response times.
- Database Optimization: Optimize your database queries and schema to ensure efficient data retrieval and manipulation.
Scenario 2: Debugging a Memory Leak
- Prompt: Your Node.js application is experiencing a gradual memory leak, causing performance degradation. How would you identify and troubleshoot the memory leak?
- Answer: Debugging memory leaks requires a systematic approach:some text
- Identify the Leak: Use profiling tools like Chrome DevTools or dedicated Node.js profiling modules to pinpoint the source of the memory leak. These tools can identify objects that are not being garbage collected properly.
- Analyze the Code: Once you've identified the suspicious code section, analyze the logic and variable usage. Common causes include unclosed event listeners, global variables holding unnecessary references, or incorrect handling of buffers.
- Refactor and Fix: Fix the identified issue by properly closing event listeners, avoiding unnecessary global variables, or managing buffer allocation and deallocation efficiently.
Scenario 3: Implementing Authentication and Authorization
- Prompt: Design a secure authentication and authorization system for your Node.js API.
- Answer: Here's a possible approach:some text
- User Registration and Login: Implement user registration with secure password hashing and storage (using bcrypt or similar libraries). Issue JWT (JSON Web Tokens) upon successful login for authentication.
- Middleware for Authorization: Create middleware that verifies the presence and validity of JWTs in incoming requests. This middleware can restrict access to specific routes based on user roles or permissions stored in the JWT payload.
- Session Management (Optional): For longer-lived sessions, consider using session management with secure storage (e.g., signed cookies with HttpOnly flag). Regularly refresh JWTs to maintain security.
**This scenario requires tailoring the answer based on specific use cases and security requirements.
Conclusion: Mastering the Node.js Interview Questions
You've embarked on a comprehensive journey, equipping yourself with the knowledge and skills to conquer your Node.js developer interview. Remember, confidence and clear communication are just as important as technical expertise. Here are some additional tips:
- Practice your answers out loud: Rehearse your responses to common interview questions(in this case: node js interview questions) to refine your explanations and delivery.
- Prepare questions for the interviewer: Demonstrating your interest in the company and role shows initiative.
- Highlight your projects and experience: Showcase your Node.js development skills by discussing relevant projects you've worked on.
- Stay calm and positive: Confidence goes a long way. Take a deep breath and approach the interview with a positive attitude.
By following these tips and leveraging the knowledge you've gained throughout this guide, you'll be well on your way to securing your dream Node.js developer position.
Congratulations! You're now prepared to shine in your Node.js developer interview.
Feeling overwhelmed by your tech job search?Weekday can help! They connect you with top companies actively seeking engineers. Leverage your network, cut through the application noise, and land your dream role faster. Sign up for Weekday today and let them streamline your job search!