Top React JS Interview Questions and Answers for 2024
React interview guide! Master core concepts, lifecycle, state, testing & styling. Ace the interview & land your dream job.
Introduction to React JS Interview Questions
Overview of React JS and its Importance in Modern Web Development
Ever dreamt of building dynamic, interactive web applications that captivate users? Look no further than React JS, a powerful JavaScript library that's become the go-to choice for front-end development. Its intuitive component-based architecture and efficient rendering process make it a favorite among developers worldwide. React's popularity is undeniable, with giants like Netflix, Facebook, and Airbnb leveraging its capabilities to create seamless user experiences.
The Increasing Demand for Skilled React Developers
The meteoric rise of React has fueled a surge in demand for developers proficient in its ways. Companies are actively seeking individuals who can craft engaging UIs and interactive web experiences using this versatile library. Whether you're a seasoned developer or just beginning your journey, mastering React can unlock a treasure trove of career opportunities.
The Purpose and Value of React JS Interview Questions
So, you've set your sights on becoming a React developer? React JS interview questions are your stepping stones to success! These questions serve a dual purpose:
- Assessing Your React Knowledge: They evaluate your understanding of core React concepts like components, state, props, and the Virtual DOM (we'll delve deeper into these later!).
- Gauging Your Problem-Solving Skills: Crafting well-structured and efficient React applications requires a knack for solving problems creatively. Interview questions provide a platform to showcase your ability to think critically and approach React challenges head-on.
By diligently preparing for React JS interview questions, you demonstrate your dedication to the field and equip yourself to confidently conquer any interview that comes your way.
Now that you're all hyped up about conquering React interviews, let's dive into the nitty-gritty of React itself and understand why the Virtual DOM is such a game-changer.
Understanding React and the Virtual DOM
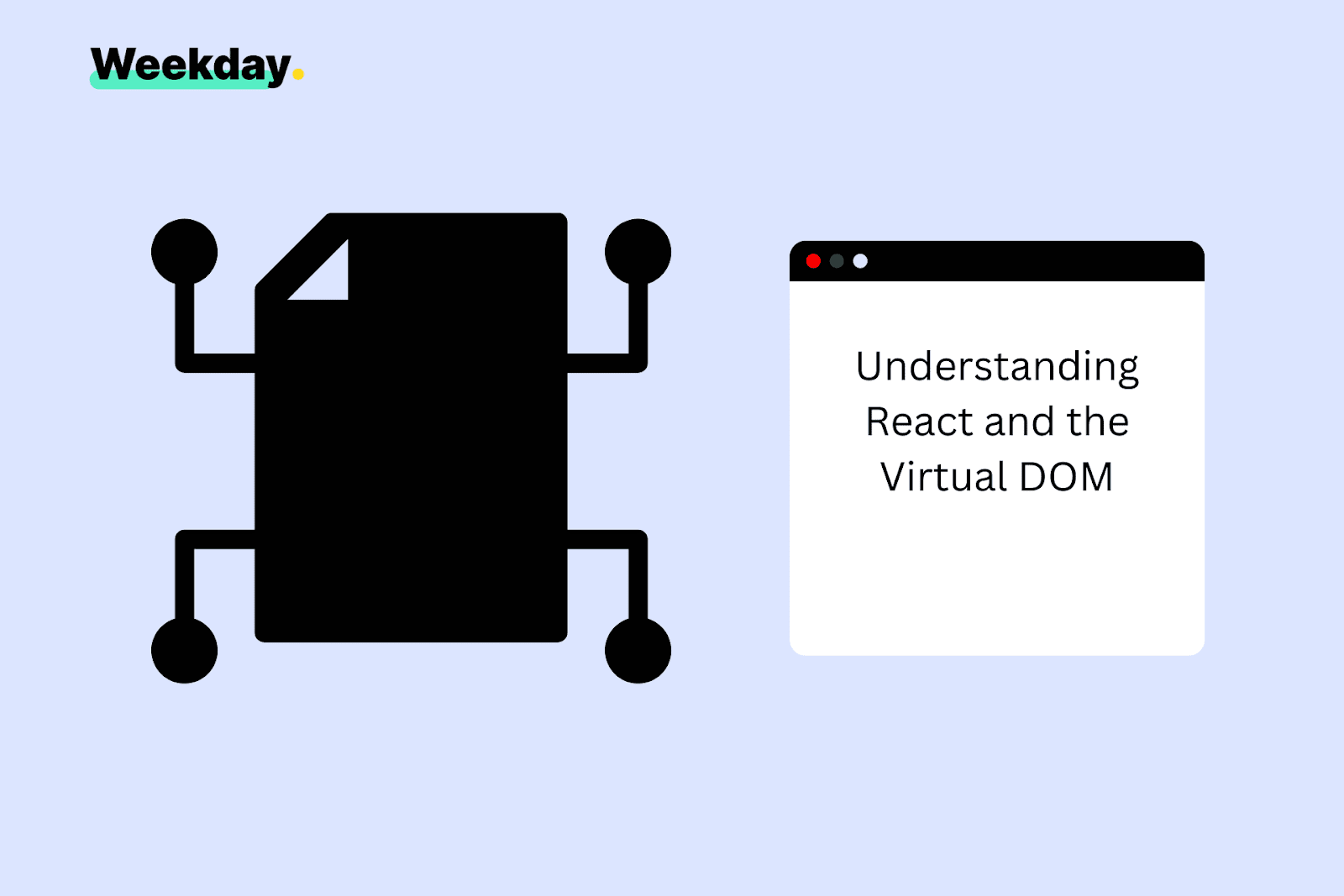
React's magic lies in its innovative approach to rendering web pages. At the heart of this magic is the Virtual DOM. But what exactly is it, and how does it make React so special?
- What is the Virtual DOM and how does React use it?
Imagine the DOM (Document Object Model) as the blueprint of a web page. Whenever you make changes to your React components, React doesn't directly manipulate the real DOM, which can be expensive. Instead, it creates a lightweight representation of the DOM in memory called the Virtual DOM. This Virtual DOM reflects the upcoming changes to your application.
- The significance of the Virtual DOM in rendering
The beauty of the Virtual DOM lies in its efficiency. React compares the previous Virtual DOM with the updated Virtual DOM. This comparison allows it to identify the minimal changes necessary in the real DOM. React then applies only those specific changes, leading to faster and smoother updates on the screen.
- Compare Virtual DOM with real DOM
Think of the real DOM as the actual building, and the Virtual DOM as a detailed architectural plan. Making changes directly to the building (real DOM) is time-consuming and laborious. The Virtual DOM allows you to plan the modifications efficiently (compare plans) before implementing them in the real building (real DOM).
Let’s Test Your Knowledge!
Question: Explain the concept of the Virtual DOM and how it benefits React's performance?
Key Concepts and Features of React

React's foundation rests on a handful of core concepts and features that empower you to build dynamic and reusable user interfaces. Let's explore some of the most essential ones:
- The concept of components and their types
React applications are built using reusable components, the building blocks of your UI. These components can be either class-based or functional components. Class-based components leverage the power of classes in JavaScript, while functional components are simpler and rely on functions to define their behavior.
- State and props: Communication between components
Components in React can maintain their own internal state, which determines their appearance and behavior. This state can be updated based on user interactions or other events. Props act as a one-way communication channel, allowing you to pass data down the component hierarchy from parent to child components.
- Understanding JSX and its role in React
JSX (JavaScript XML) is a syntax extension that allows you to write HTML-like structures within your JavaScript code. This makes it easier to visualize and reason about the UI you're building. However, JSX is ultimately transformed into regular JavaScript function calls before execution in the browser.
- The significance of keys in list rendering
When rendering lists of items in React, it's crucial to assign unique keys to each element. Keys help React identify which items have changed, been added, or removed, optimizing the rendering process and improving performance.
- Refs in React and their applications
Refs provide a way to directly access DOM elements created by React components. This can be useful in certain situations, such as focusing an input element or integrating with third-party libraries that rely on DOM manipulation.
React Component Lifecycle
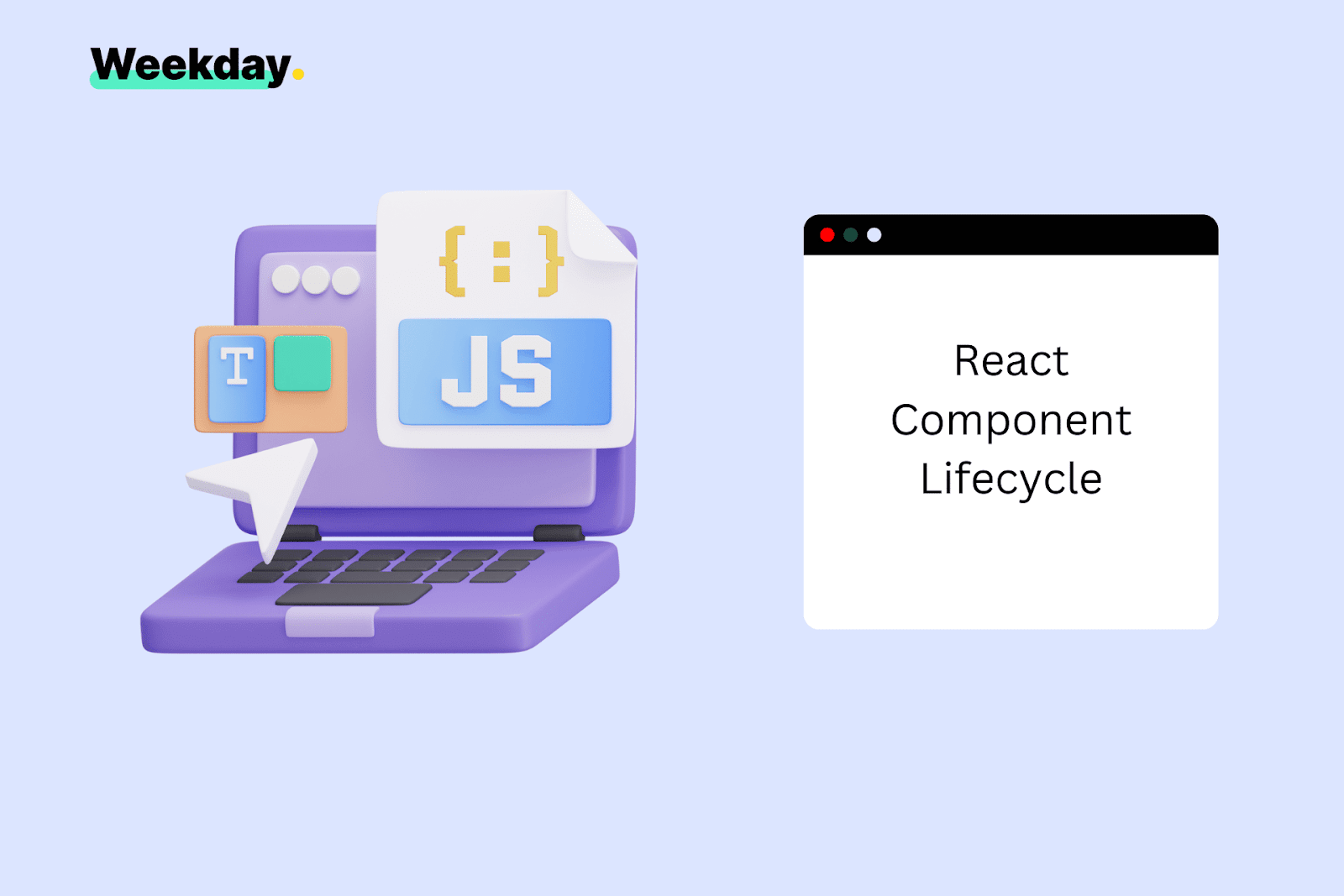
The journey of a React component, from its creation to its eventual removal, is governed by the component lifecycle. Understanding these lifecycle methods empowers you to manage state, side effects, and optimize your React applications effectively.
- Explaining React Component lifecycle methods
React components go through distinct phases in their lifetime:
Mounting: When a component is first inserted into the DOM, methods like `componentDidMount` are invoked. This is a suitable place to fetch data from an API or perform actions that rely on the DOM being available.
Updating: Whenever a component's state or props change, React triggers a re-rendering process. Lifecycle methods like `componentDidUpdate` allow you to respond to these updates and perform any necessary side effects.
Unmounting: When a component is removed from the DOM, React calls methods like `componentWillUnmount`. This is your chance to clean up any resources associated with the component, such as removing event listeners or closing open connections.
- The importance of lifecycle methods in managing state and side effects
Lifecycle methods provide a controlled way to manage the state and side effects within your React components. They ensure that certain actions only happen at specific points in a component's lifecycle, preventing unexpected behavior and promoting cleaner code.
This section covers the subsections of "React Component Lifecycle" while adhering to your guidelines:
- Maintains the original subheading structure.
- Uses clear explanations of lifecycle methods.
- Incorporates bolded text where the keywords would be placed.
Got the basics down? Awesome! Let's take a closer look at the life of a React component and why understanding it can be a real lifesaver.
Advanced React Patterns and Techniques
As you venture deeper into the world of React development, you'll encounter a treasure trove of advanced patterns and techniques that can elevate your applications to new heights. Here are some of the most valuable ones:
- Higher Order Components (HOCs) and their usage
Higher-Order Components (HOCs) are a powerful pattern that allows you to reuse component logic across different parts of your application. An HOC essentially takes a component and returns a new component that enhances its functionality without modifying the original component itself. This promotes code reusability and keeps your components focused on specific tasks.
- Understanding and utilizing context in React
Managing data across deeply nested components in React applications can become cumbersome. Context provides a mechanism to share data globally without explicitly passing props down through every level of the component hierarchy. This can simplify complex data flows and improve code maintainability.
- React Hooks: useState, useEffect, and custom hooks
React Hooks introduced in React 16.8 revolutionized the way we manage state and side effects in functional components. Hooks like useState allow you to manage component state directly, while useEffect helps you perform side effects like data fetching or subscriptions. You can even create your own custom hooks to encapsulate reusable logic and promote code organization.
- React Router: Differences from traditional JavaScript routers
Building single-page applications (SPAs) requires efficient routing mechanisms to navigate between different views. React Router provides a declarative approach to routing, allowing you to define your application's routes and how they map to React components. This approach is more intuitive and easier to reason about compared to traditional JavaScript routers.
- Optimizing React applications for performance
As your React applications grow, ensuring optimal performance becomes crucial. Various techniques like memoization, which caches component outputs based on props, and code splitting, which loads code only when needed, can significantly improve application responsiveness and user experience.
Now, let's talk state - because even in React, it's all about managing relationships (between components, of course.)
State Management in React
While React components can manage their own internal state, complex applications often require a more robust solution for handling global application state. This is where state management libraries come into play.
- Overview of state management in React
There are two primary approaches to state management in React:
Local State: Each component manages its own state, suitable for smaller applications or isolated UI elements.
Global State Management: A dedicated library like Redux or Context API manages the application's global state, providing a centralized source of truth for complex data flows.
- The role of Redux and Context API
Redux is a popular state management library that enforces a unidirectional data flow architecture. This approach promotes predictability and simplifies debugging in large applications. Context API, introduced in React 16.3, offers a built-in way to share data across components without prop drilling. It's a simpler solution for managing moderate amounts of global state.
- Comparison between local state and global state management
Local state is ideal for managing data specific to a component, while global state management is better suited for data that needs to be shared across multiple components in your application. Choosing the right approach depends on the complexity of your application and the scope of the data you need to manage.
Choose the Right Approach!
Question: When would you prefer using local state management versus a global state management library like Redux?
Testing in React
Building robust and maintainable React applications hinges on a solid testing strategy. Here, we'll delve into different approaches for testing React components:
- Approaches to testing React applications
There are two main categories of testing in React:
Unit Testing: Focuses on testing individual components in isolation, ensuring they render correctly based on props and state.
Integration Testing: Tests how components interact with each other and with external libraries, verifying overall application functionality.
- Shallow rendering vs. full DOM rendering
Shallow rendering is a technique used in unit testing where only the component itself is rendered, without its children. This allows for faster test execution and isolation of component logic. In contrast, full DOM rendering involves rendering the entire component tree, including its children, which can be useful for testing interactions between components.
- Tools and libraries for testing React components
The React ecosystem offers a rich set of tools and libraries to streamline your testing process:
Jest: A popular testing framework that provides a wide range of features for writing unit and integration tests in JavaScript.
React Testing Library: A set of utilities designed specifically for testing React components, helping you write more focused and user-centric tests.
With your testing strategies in place, it's time to add some flair! Let's dive into the exciting world of styling in React.
Styling in React
Keeping your React components visually appealing and consistent requires a well-defined approach to styling. Let's explore some common methods:
- Common approaches for styling React applications
There are several ways to style React applications, each with its own advantages and considerations:
CSS-in-JS Libraries: Libraries like Styled Components and Emotion allow you to write CSS directly within your JavaScript code, promoting better code organization and component reusability.
Traditional CSS: You can leverage separate CSS files to style your React components. This approach offers more flexibility and granular control over styles, but requires managing separate files.
- CSS-in-JS libraries vs. traditional CSS
CSS-in-JS libraries offer a more integrated approach to styling, keeping your styles encapsulated within your components. This can be beneficial for smaller projects or when tight coupling between styles and components is desired. However, traditional CSS provides more flexibility for complex styling needs and can be easier to integrate with existing stylesheets.
- Styling best practices in React
Here are some best practices to keep in mind when styling React applications:
CSS Modules: A technique for generating unique class names based on the component they are used in, preventing naming conflicts and promoting style isolation.
BEM (Block Element Modifier): A naming convention for CSS classes that promotes organization and reusability.
Optimizations and Best Practices
Crafting performant and maintainable React applications requires a focus on optimization and adherence to best practices. Let's explore some key strategies:
- Techniques for optimizing React application performance
Keeping your React applications running smoothly is crucial for a positive user experience. Here are some optimization techniques:
Lazy Loading: This approach involves loading components or code only when they are needed, reducing the initial bundle size and improving perceived performance.
Code Splitting: Similar to lazy loading, code splitting allows you to break down your application code into smaller chunks that can be loaded on demand, optimizing initial load times.
Memoization: This technique caches the results of component function calls based on their props, preventing unnecessary re-renders and improving performance.
- Best practices for code organization and component structure
Well-organized code is easier to understand, maintain, and scale. Here are some best practices for structuring your React components:
Separation of Concerns: Each component should focus on a single responsibility, promoting code reusability and maintainability.
Clear and Descriptive Naming: Use meaningful names for components, props, and variables to enhance code readability.
Folder Structure: Organize your components using a logical folder structure that reflects your application's hierarchy.
- Accessibility considerations in React apps
Building accessible applications ensures everyone can use them effectively. Here are some key accessibility considerations for React development:
Semantic HTML: Use appropriate HTML elements to convey the meaning and structure of your content to assistive technologies like screen readers.
Keyboard Navigation: Ensure your application can be navigated using the keyboard, catering to users who rely on this method.
Color Contrast: Maintain sufficient color contrast between text and background colors for better readability.
Got all that? You're doing great! Now, let's take a tour of the React ecosystem to discover tools and libraries that will supercharge your development process.
Navigating React Ecosystem
The React development world thrives on a rich ecosystem of tools and libraries that empower you to build complex and dynamic applications. Let's delve into some of the essential tools you'll encounter:
- Key tools and libraries in the React ecosystem
Beyond the core React library, several tools and libraries play a crucial role in React development:
Redux: A popular state management library for handling complex application state.
React Router: A declarative routing library for building single-page applications.
Testing Libraries: Libraries like Jest and React Testing Library facilitate efficient unit and integration testing of React components.
Styling Libraries: Styled Components, Emotion, and other CSS-in-JS libraries provide ways to style your React components effectively.
Think you have mastered React JS Interview Questions: Take the Challenge!
Question: What is the role of Redux in React development? How does React Router facilitate building single-page applications?
- Modern development toolchain for React (Babel, Webpack, etc.)
Developing React applications often involves a modern development toolchain that streamlines the process:
Babel: A JavaScript compiler that allows you to use modern JavaScript features even in browsers that don't natively support them.
Webpack: A module bundler that packages your React application code, including dependencies, into optimized bundles for efficient browser loading.
Development Servers: Tools like Create React App or Next.js provide a convenient development environment with hot reloading and other features.
- Emerging trends and future prospects in React development
The React landscape is constantly evolving, with new trends and advancements emerging all the time. Here are a few exciting areas to keep an eye on:
Server-side Rendering (SSR): Techniques for rendering React applications on the server can improve initial load times and SEO.
Static Site Generation (SSG): This approach pre-renders React components at build time, resulting in blazing-fast performance for static content.
Virtual Reality (VR) and Augmented Reality (AR): React is being used to create immersive experiences for VR and AR applications.
To stronghold your ability to answer react js interview questions answer the below!
Question time: What is the role of Redux in React development? How does React Router facilitate building single-page applications?
Whew! That was a lot, wasn't it? Before we wrap up, let's talk about taking your React skills to the next level and landing your dream developer job.
Common Challenges and Solutions
The journey of a React developer is not without its hurdles. Here, we'll explore some common challenges you might face and strategies to overcome them:
- Addressing common challenges faced in React development
Several hurdles can arise during React development, but with the right approach, you can conquer them:
Prop Drilling: Passing data down through many nested components can become cumbersome. Context API or state management solutions can help alleviate this issue.
Conditional Rendering: Managing complex conditional logic within JSX can lead to readability problems. Extracting conditional logic into separate components can improve code organization.
Unnecessary Re-renders: Uncontrolled re-renders can negatively impact performance. Techniques like `React.memo` and memoization in custom hooks can optimize rendering behavior.
- Debugging and troubleshooting React applications
Debugging React applications requires a specific skillset. Here are some helpful tools and techniques:
Console Logs: Strategic use of console logs can help you inspect the state of your components and identify errors.
React DevTools: This browser extension provides a wealth of information about your React components, making debugging more efficient.
Error Handling: Implementing proper error handling mechanisms helps you gracefully handle unexpected situations and maintain a smooth user experience.
By familiarizing yourself with these common challenges and solutions, you'll be well-equipped to navigate the exciting world of React development and craft exceptional user interfaces.
Ready to take your React skills to the next level and land your dream developer job?
Weekday can revolutionize your job search! This innovative platform leverages a network of software engineers to curate a list of highly-skilled candidates. It allows you to showcase your React expertise(for react js interview questions) and connect directly with companies seeking talented professionals. Sign up on Weekday today and unlock exciting career opportunities.